This is the second post of the tutorial series called Node Hero - in these chapters you can learn how to get started with Node.js and deliver software products using it. Planned and past chapters:
- Getting started with Node.js
- Using NPM [you are reading it now]
- Understanding async programming
- Your first Node.js server
- Node.js Database Tutorial
- Node.js request module tutorial
- Node.js project structure tutorial
- Node.js authentication using Passport.js
- Node.js unit testing tutorial
- Debugging Node.js applications
- Node.js Security Tutorial
- How to Deploy Node.js Applications
- Monitoring Node.js Applications
In this chapter, you'll learn what NPM is and how to use it. Let's get started!
NPM in a Nutshell
NPM is the package manager used by Node.js applications - you can find a ton of modules here, so you don't have to reinvent the wheel. It is like Maven for Java or Composer for PHP. There are two primary interfaces you will interact with - the NPM website and the NPM command line toolkit.
Both the website and the CLI uses the same registry to show modules and search for them.
The Website
The NPM website can be found at https://npmjs.com. Here you can sign up as a new user or search for packages.
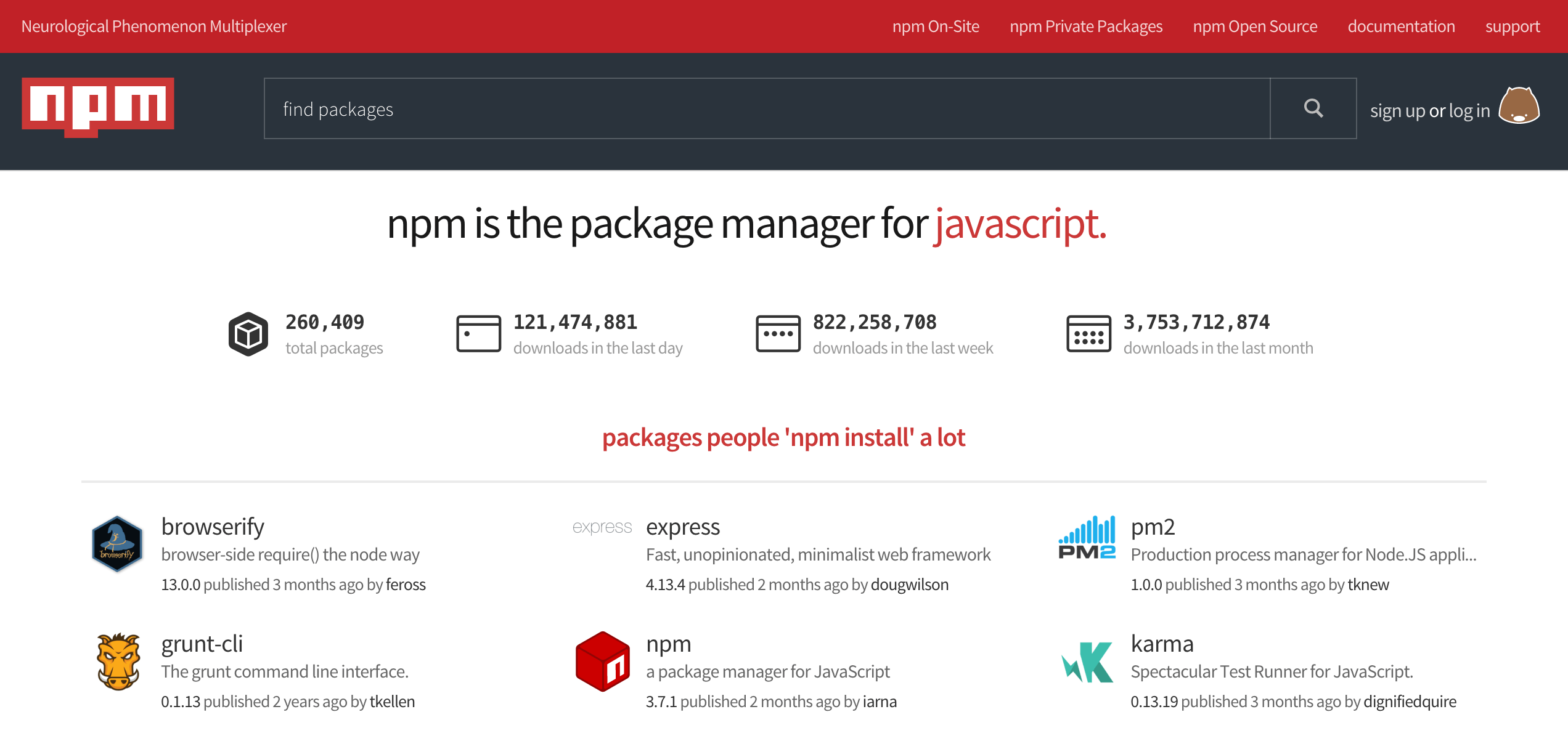
The Command Line Interface
To run the CLI you can run it simply with:
npm
Note, that NPM is bundled with the Node.js binary, so you don't have to install it - however, if you want to use a specific npm version, you can update it. If you want to install npm version 3, you can do that with:
npm install npm@3 -g
.Using NPM: Tutorial
You have already met NPM in the last article on Getting started with Node.js when you created the
package.json
file. Let's extend that knowledge!Adding Dependencies
In this section you are going to learn how to add runtime dependencies to your application.
Once you have your
package.json
file you can add dependencies to your application. Let's add one! Try the following:npm install lodash --save
With this single command we achieved two things: first of all,
lodash
is downloaded and placed into the node_modules
folder. This is the folder where all your external dependencies will be put. Usually, you don't want to add this folder to your source control, so if you are using git make sure to add it to the .gitignore
file.
This can be a good starting point for your
.gitignore
Let's take a look at what happened in the
package.json
file! A new property called dependencies
have appeared:"dependencies": {
"lodash": "4.6.1"
}
This means that
lodash
with version 4.6.1
is now installed and ready to be used. Note, that NPM follows SemVer to version packages.Given a version number MAJOR.MINOR.PATCH, increment the MAJOR version when you make incompatible API changes, MINOR version when you add functionality in a backwards-compatible manner, and PATCH version when you make backwards-compatible bug fixes. For more information: http://semver.org/
As
lodash
is ready to be used, let's see how we can do so! You can do it in the same way you did it with your own module except now you don't have to define the path, only the name of the module:// index.js
const _ = require('lodash')
_.assign({ 'a': 1 }, { 'b': 2 }, { 'c': 3 });
// → { 'a': 1, 'b': 2, 'c': 3 }
Adding Development Dependencies
In this section you are going to learn how to add build-time dependencies to your application.
When you are building web applications, you may need to minify your JavaScript files, concatenating CSS files and so on. The modules that will do it will be only ran during the building of the assets, so the running application doesn't need them.
You can install such scripts with:
npm install mocha --save-dev
Once you do that, a new section will appear in your
package.json
file called devDependencies
. All the modules you install with --save-dev
will be placed there - also, they will be put in the very same node_modules
directory.NPM Scripts
NPM script is a very powerful concept - with the help of them you can build small utilities or even compose complex build systems.
The most common ones are the
start
and the test
scripts. With the start
you can define how one should start your application, while test
is for running tests. In your package.json
they can look something like this: "scripts": {
"start": "node index.js",
"test": "mocha test",
"your-custom-script": "echo npm"
}
Things to notice here:
start
: pretty straightforward, it just describes the starting point of your application, it can be invoked withnpm start
test
: the purpose of this one is to run your tests - one gotcha here is that in this casemocha
doesn't need to be installed globally, as npm will look for it in thenode_modules/.bin
folder, and mocha will be placed there as well. It can be invoked with:npm test
.your-custom-script
: anything that you want, you can pick any name. It can be invoked withnpm run your-custom-script
- don't forget therun
part!
Scoped / Private Packages
Originally NPM had a global shared namespace for module names - with more than 250.000 modules in the registry most of the simple names are already taken. Also, the global namespace contains public modules only.
NPM addressed this problem with the introduction of scoped packages. Scoped packages has the following naming pattern:
@myorg/mypackage
You can install scoped packages the same way as you did before:
npm install @myorg/mypackage --save-dev
It will show up in your
package.json
in the following way:"dependencies": {
"@myorg/mypackage": "^1.0.0"
}
Requiring scoped packages works as expected:
require('@myorg/mypackage')
No comments:
Post a Comment